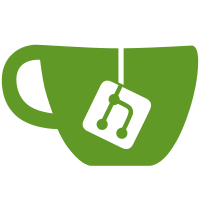
- renamed library - added support for saving loading current game - added (still crappy) nonogram solver - some code cleanups
80 lines
1.8 KiB
C++
80 lines
1.8 KiB
C++
#ifndef LIBQCROSS_CNONOGRAMSOLVER_H
|
|
#define LIBQCROSS_CNONOGRAMSOLVER_H
|
|
|
|
#include <QObject>
|
|
|
|
namespace libqnono {
|
|
class CNonogram;
|
|
|
|
class CNonogramSolver : public QObject {
|
|
Q_OBJECT
|
|
public:
|
|
CNonogramSolver(QObject * parent = 0);
|
|
~CNonogramSolver();
|
|
|
|
void setNonogram(CNonogram * nonogram);
|
|
public slots:
|
|
bool solve();
|
|
signals:
|
|
void markRequested(int x, int y, int type);
|
|
protected:
|
|
enum MarkerType {CMT_UNMARKED = 0, CMT_MARKED = 1, CMT_CROSSED = 2, CMT_NONE = 3};
|
|
|
|
struct NumberOverlay {
|
|
int borderLeft;
|
|
int borderRight;
|
|
|
|
quint16 entry;
|
|
bool finished;
|
|
};
|
|
|
|
struct LineOverlay {
|
|
NumberOverlay * numbers;
|
|
int numbersSize;
|
|
|
|
bool dirty;
|
|
|
|
inline int borderLeftDef(int index, int def) {
|
|
return (index > -1 && index < numbersSize) ? numbers[index].borderLeft : def;
|
|
}
|
|
|
|
inline int borderRightDef(int index, int def) {
|
|
return (index > -1 && index < numbersSize) ? numbers[index].borderRight : def;
|
|
}
|
|
|
|
LineOverlay() : numbers(NULL), dirty(false) {}
|
|
~LineOverlay() { if (numbers) delete[] numbers; }
|
|
};
|
|
|
|
CNonogram * m_Nonogram;
|
|
|
|
LineOverlay * m_RowsOverlay;
|
|
LineOverlay * m_ColumnsOverlay;
|
|
|
|
int ** m_OverlayData;
|
|
|
|
void cleanup();
|
|
void prepare();
|
|
|
|
inline void mark(int x, int y, int marker);
|
|
inline void fillRow(int index, int marker);
|
|
inline void fillColumn(int index, int marker);
|
|
|
|
void printOverlays();
|
|
bool solveLine_ng(int index, bool isRow);
|
|
|
|
inline bool solveLine(int index, bool isRow);
|
|
inline bool fillGaps(int index, bool isRow);
|
|
inline void prepareBorders(LineOverlay * overlay);
|
|
inline void updateBorders(int index, bool isRow);
|
|
|
|
void updateBorders();
|
|
|
|
inline int safeLength(NumberOverlay * overlay) {
|
|
return (overlay->entry * 2) + overlay->borderLeft - overlay->borderRight;
|
|
}
|
|
};
|
|
}
|
|
|
|
#endif
|